October 27, 2017
Two fundamental abstraction facilities
–Process abstraction
- Emphasized from early days
- Discussed in this chapter
–Data abstraction
- Emphasized in the1980s
- Discussed at length in Chapter 11
Fundamentals of Subprograms
- Each subprogram has a single entry point
- The calling program is suspended during execution of the called subprogram
- Control always returns to the caller when the called subprogram’s execution terminates
Local Referencing Environments
- Local variables can be stack-dynamic
–Advantages
- Support for recursion
- Storage for locals is shared among some subprograms
–Disadvantages
- Allocation/de-allocation, initialization time
- Indirect addressing
- Subprograms cannot be history sensitive
- Local variables can be static
–Advantages and disadvantages are the opposite of those for stack-dynamic local variables
Semantic Models of Parameter Passing
- In mode
- Out mode
- Inout mode
Models of Parameter Passing
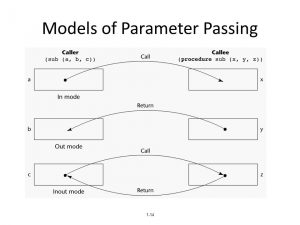
Models of Parameter Passing
Pass-by-Value (In Mode)
- The value of the actual parameter is used to initialize the corresponding formal parameter
–Normally implemented by copying
–Can be implemented by transmitting an access path but not recommended (enforcing write protection is not easy)
–Disadvantages (if by physical move): additional storage is required (stored twice) and the actual move can be costly (for large parameters)
–Disadvantages (if by access path method): must write-protect in the called subprogram and accesses cost more (indirect addressing)
Pass-by-Result (Out Mode)
- When a parameter is passed by result, no value is transmitted to the subprogram; the corresponding formal parameter acts as a local variable; its value is transmitted to caller’s actual parameter when control is returned to the caller, by physical move
–Require extra storage location and copy operation
- Potential problems:
– sub(p1, p1); whichever formal parameter is copied back will represent the current value of p1
–sub(list[sub], sub); Compute address of list[sub] at the beginning of the subprogram or end?
Pass-by-Value-Result (inout Mode)
- A combination of pass-by-value and pass-by-result
- Sometimes called pass-by-copy
- Formal parameters have local storage
- Disadvantages:
–Those of pass-by-result
–Those of pass-by-value
Pass-by-Reference (Inout Mode)
- Pass an access path
- Also called pass-by-sharing
- Advantage: Passing process is efficient (no copying and no duplicated storage)
- Disadvantages
–Slower accesses (compared to pass-by-value) to formal parameters
–Potentials for unwanted side effects (collisions)
–Unwanted aliases (access broadened)
fun(total, total); fun(list[i], list[j]; fun(list[i], i);
Pass-by-Name (Inout Mode)
- By textual substitution
- Formals are bound to an access method at the time of the call, but actual binding to a value or address takes place at the time of a reference or assignment
- Allows flexibility in late binding
- Implementation requires that the referencing environment of the caller is passed with the parameter, so the actual parameter address can be calculated
Implementing Parameter-Passing Methods
Function header: void sub(int a, int b, int c, int d)
Function call in main: sub(w, x, y, z)
(pass w by value, x by result, y by value-result, z by reference)
Design Considerations for Parameter Passing
- Two important considerations
–Efficiency
–One-way or two-way data transfer
- But the above considerations are in conflict
–Good programming suggest limited access to variables, which means one-way whenever possible
–But pass-by-reference is more efficient to pass structures of significant size
Calling Subprograms Indirectly
- Usually when there are several possible subprograms to be called and the correct one on a particular run of the program is not know until execution (e.g., event handling and GUIs)
- In C and C++, such calls are made through function pointers
Overloaded Subprograms
- An overloaded subprogram is one that has the same name as another subprogram in the same referencing environment
–Every version of an overloaded subprogram has a unique protocol
- C++, Java, C#, and Ada include predefined overloaded subprograms
- In Ada, the return type of an overloaded function can be used to disambiguate calls (thus two overloaded functions can have the same parameters)
- Ada, Java, C++, and C# allow users to write multiple versions of subprograms with the same name
Generic Subprograms
- A generic or polymorphic subprogram takes parameters of different types on different activations
- Overloaded subprograms provide ad hoc polymorphism
- Subtype polymorphism means that a variable of type T can access any object of type T or any type derived from T (OOP languages)
- A subprogram that takes a generic parameter that is used in a type expression that describes the type of the parameters of the subprogram provides parametric polymorphism
– A cheap compile-time substitute for dynamic binding
User-Defined Overloaded Operators
- Operators can be overloaded in Ada, C++, Python, and Ruby
- A Python example
def __add__ (self, second) :
return Complex(self.real + second.real,
self.imag + second.imag)
Use: To compute x + y, x.__add__(y)
Closures
- A closure is a subprogram and the referencing environment where it was defined
–The referencing environment is needed if the subprogram can be called from any arbitrary place in the program
–A static-scoped language that does not permit nested subprograms doesn’t need closures
–Closures are only needed if a subprogram can access variables in nesting scopes and it can be called from anywhere
–To support closures, an implementation may need to provide unlimited extent to some variables (because a subprogram may access a nonlocal variable that is normally no longer alive)
Coroutines
- A coroutine is a subprogram that has multiple entries and controls them itself – supported directly in Lua
- Also called symmetric control: caller and called coroutines are on a more equal basis
- A coroutine call is named a resume
- The first resume of a coroutine is to its beginning, but subsequent calls enter at the point just after the last executed statement in the coroutine
- Coroutines repeatedly resume each other, possibly forever
- Coroutines provide quasi-concurrent execution of program units (the coroutines); their execution is interleaved, but not overlapped